Custom Functions
With Custom Functions, you can easily extend Transcend's integration functionality to connect to any API or home-grown system.
Custom Functions are serverless functions that execute on Sombra™ as part of a workflow. Unlike webhooks, Custom Functions require zero infrastructure to set up. Just write your code, and publish.
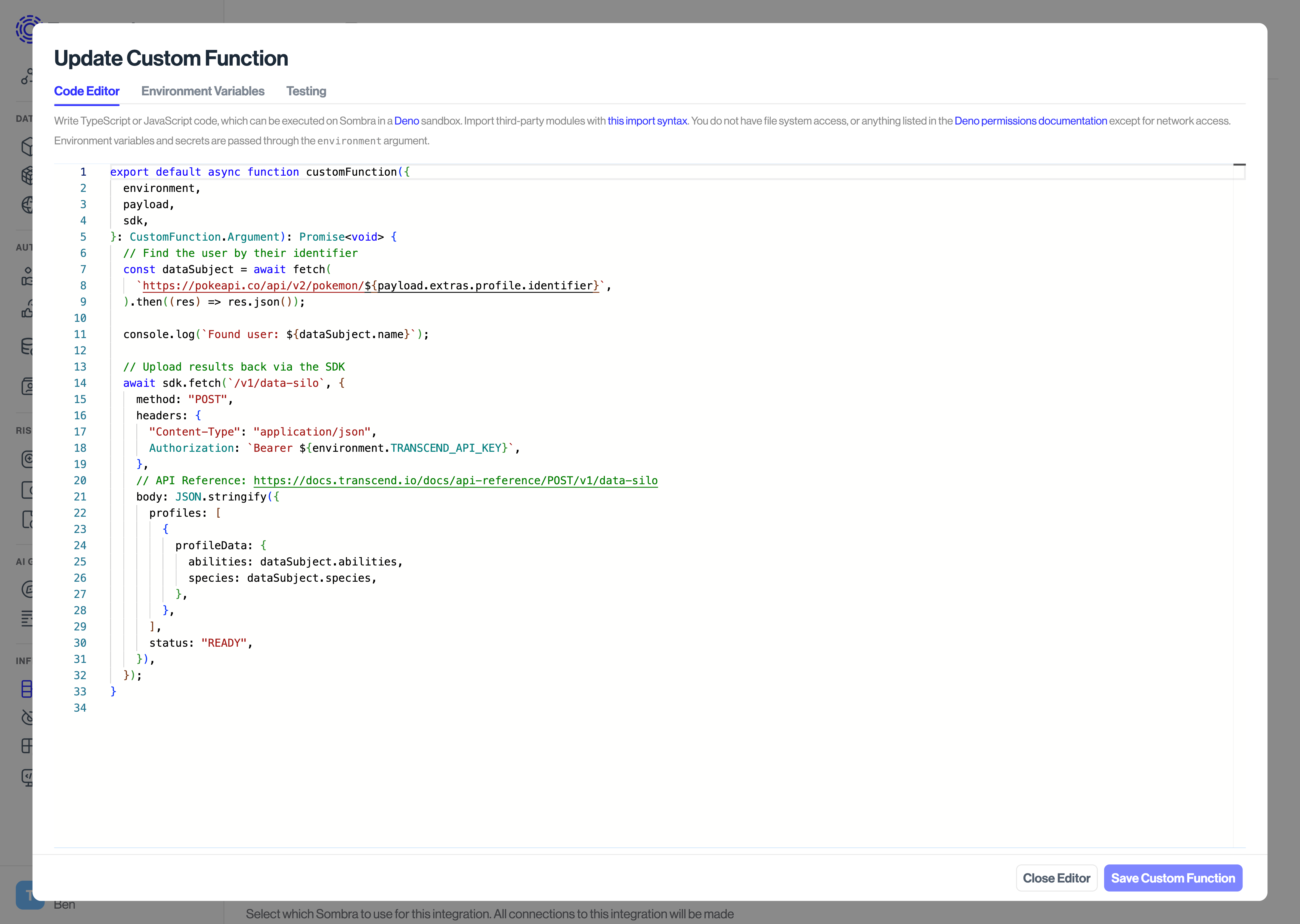
Note: Custom Functions are currently in beta.
To use them during the beta period, you must have a self-hosted Sombra cluster. If you wish to use Custom Functions without a self-hosted Sombra cluster, please contact your account representative.
Custom Functions are written in TypeScript, and execute in a Deno runtime. They run in the context of a Transcend workflow, and have access to the workflow's context.
export default async function customFunction({ environment, payload, sdk, }: CustomFunction.Argument): Promise<void> { console.log('Hello, world!'); }
- The default export is the main function that will be executed.
- You can also write code outside of the main function—the whole file will be executed.
- You can rename the function, so long as it has
export default async function
.
- You have full access to third-party libraries.
Deno has broad support for Web APIs, including fetch
. We recommend using fetch
to make calls to your services.
const response = await fetch('https://api.example.com/opt-out', { method: 'POST', headers: { 'Content-Type': 'application/json', Authorization: `Bearer ${environment.API_KEY}`, }, body: JSON.stringify({ key: 'value' }), });
You can import modules from a remote registry and Deno will automatically download them on the first run, and cache them for future runs. You can read more about Deno's import system here.
import { say } from 'jsr:@morinokami/deno-says/say'; export default async function customFunction({ environment, payload, sdk, }: CustomFunction.Argument): Promise<void> { say('Hello from Deno!'); }
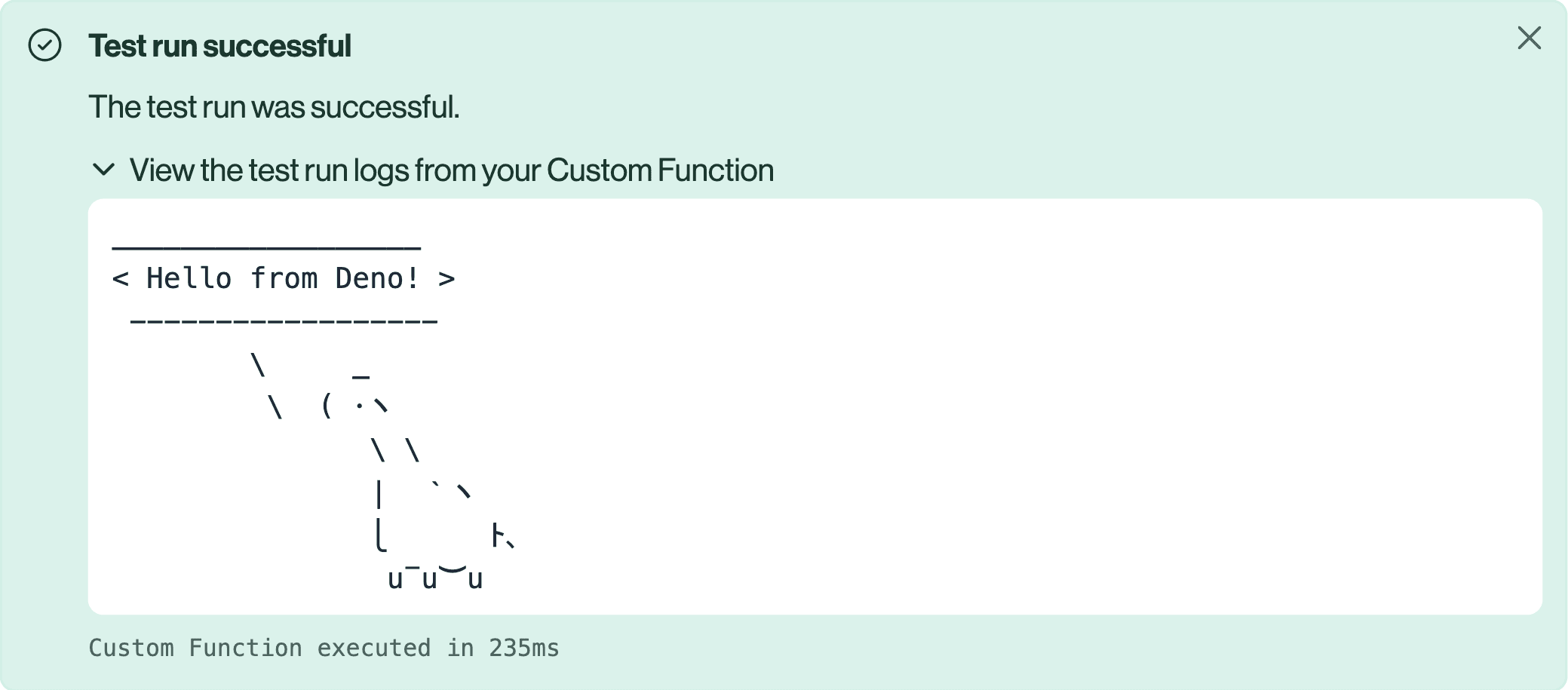
The Custom Function editor supports IntelliSense, which will show you the Custom Function argument types, return types, and the globals available in the Deno runtime.
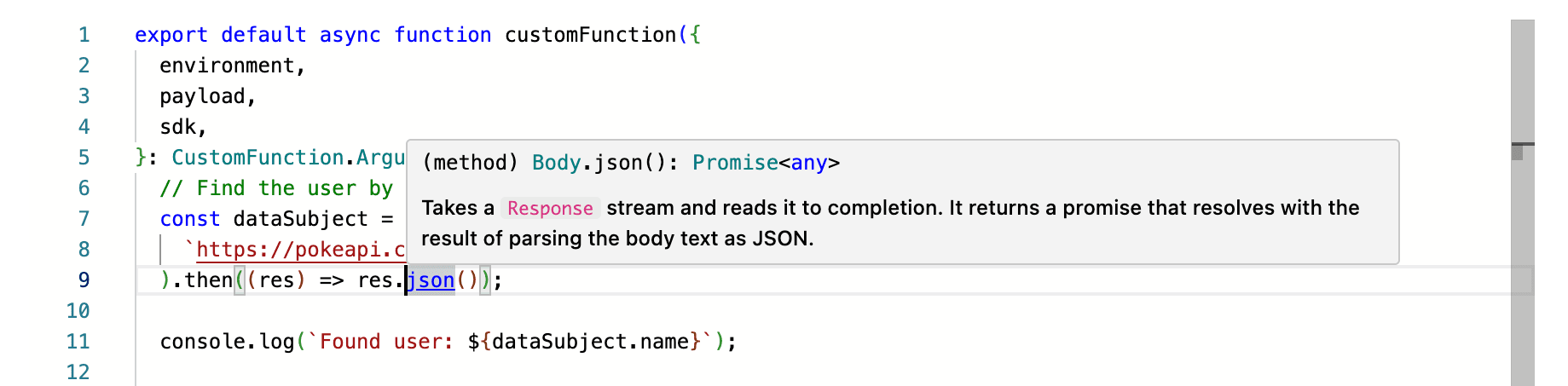
There are three arguments to your Custom Function:
environment
: The environment variables for the workflow. These are secret variables set by you in the Environment Variables tab of the Custom Function.payload
: The payload the workflow context, which contains information such as the action that triggered the workflow (such as an access request, or a contact opt-out), the user's identifiers, and more. It is identical to the "New Privacy Request Job" request body. It is also fully typed, so you can use IntelliSense to reference the documentation.sdk
: An SDK for interacting with the Sombra API, such as to upload data to a system. This is a simple wrapper aroundfetch
, which abstracts away a few details, such as the URL for the Sombra API, and authentication.
The Environment Variables tab is where you should store API keys and other sensitive configuration parameters. You should not store secret values directly in the Custom Function code. These values are securely stored by Sombra, and Transcend's cloud does not have access to them. When you set these values through your the Admin Dashboard, they are uploaded to Sombra via an end-to-end encrypted connection.
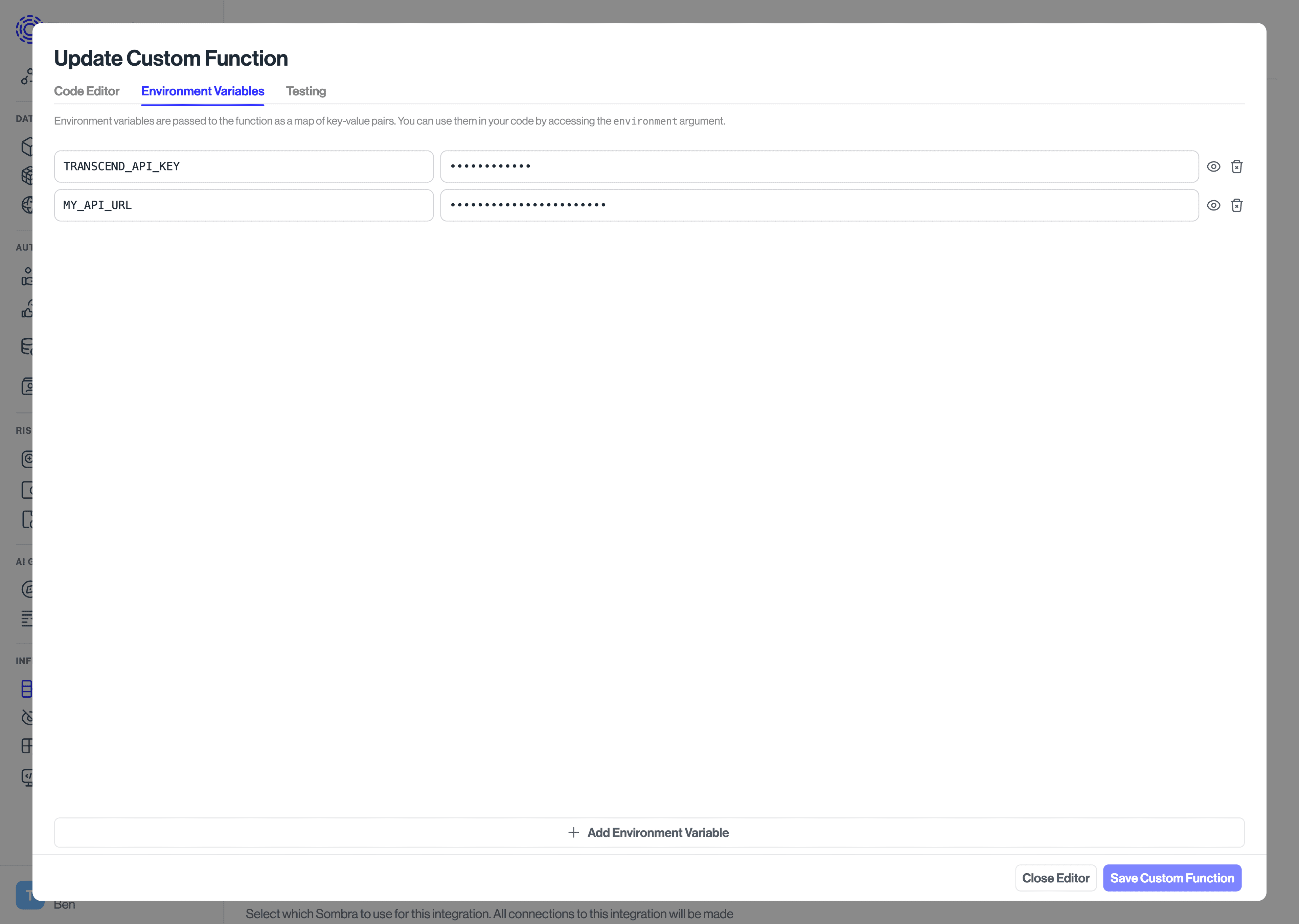
In the Testing tab, you can execute your Custom Function with a test payload, which will be passed to the payload
argument. Click Send Test Request to execute the Custom Function. The Custom Function will be executed in a new sandboxed process, and the log output will be shown in the console. To log output to the console, use console.log
.
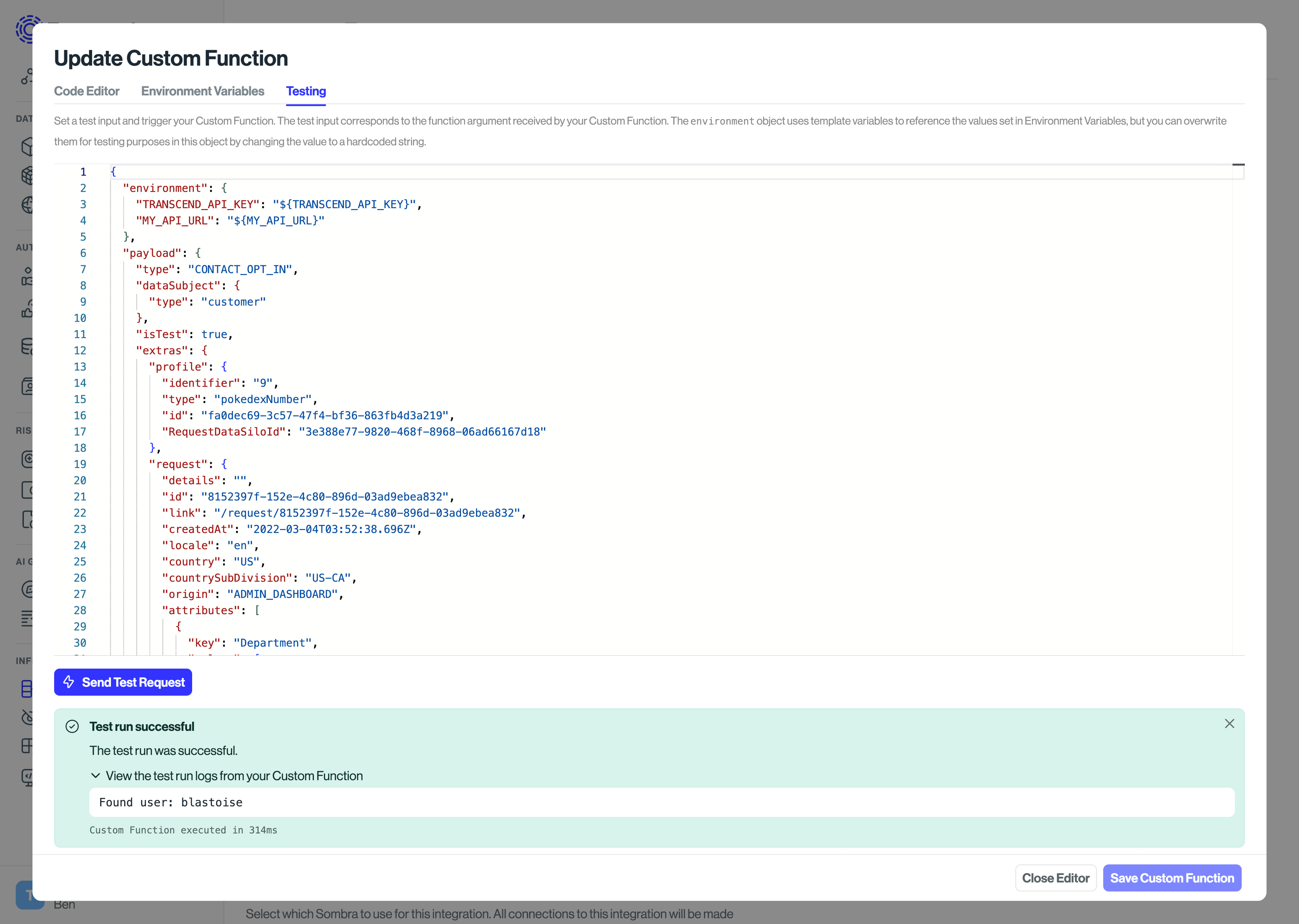
-
Navigate to Integrations
-
Add a "Server Webhook" integration (while Custom Functions are in beta, they will live alongside webhooks in the integrations page)
-
On the Connection tab of the integration, under Connection Strategy click the dropdown and switch from "Send a webhook" to "Run a Custom Function".
-
Click "Update Custom Function".
- The maximum runtime for your Custom Function is limited to 10 seconds. You can increase this if you are self-hosting Sombra.
- You do not have access to the file system.
- You do not have access to system environment variables (however, you can set your own secret variables in the Custom Function editor).
- You cannot spawn child processes.
- You cannot access system information.
- You cannot call foreign functions (e.g., a C++ library).
Out of this list of Deno permissions, only Network Access is granted.
- No two Transcend customers run Custom Functions on the same hardware.
- Each Custom Function invocation is run in a new sandboxed process.